Data (1D)¶
Importing EPRpy¶
[17]:
import eprpy as epr
Loading data¶
[18]:
tempo = epr.load('tempo.DSC')
[19]:
fig,ax=tempo.plot()
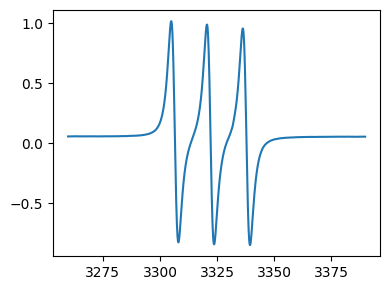
[6]:
type(tempo) # tempo is an EprData object
[6]:
eprpy.loader.EprData
Plotting¶
Every
EprData
object has a plot method for making quick plots for inspection.
[4]:
fig,ax = tempo.plot()
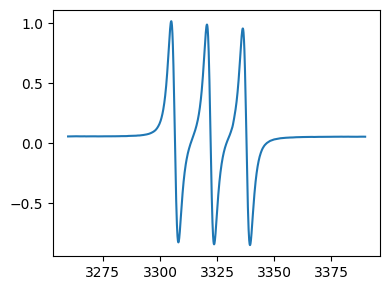
Formatting plots¶
[5]:
ax.set_xlabel('Magnetic Field [Gauss]') # set x axis label
fig
[5]:
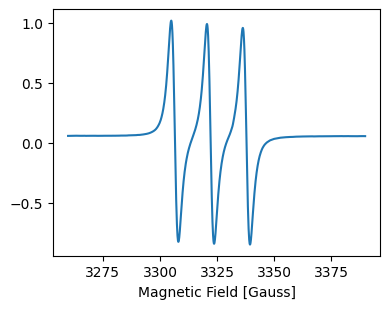
Use attributes directly to plot¶
Alternatively, plots can be made directly using matplotlib and
EprData
attributes with full control over formatting.
[6]:
import matplotlib.pyplot as plt
fig,ax = plt.subplots(figsize=(8,4))
ax.plot(tempo.x/10,tempo.data,color='black') # convert Gauss to mT
ax.set_xlabel('Magnetic Field [mT]') # set x axis label
_=ax.set_yticks([]) # hide intensity
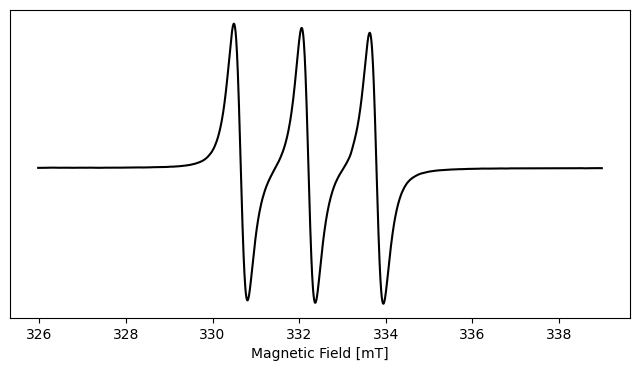
Plot on the g axis¶
If the one of the axis is magnetic field, the g values are calculated internally and can be used for plotting as shown below.
[21]:
fig,ax = tempo.plot(g_scale=True)
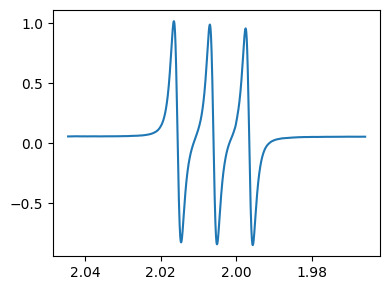
Operations on Data¶
Scaling data¶
[7]:
# import EPRpy
import eprpy as epr
# load data
tempo = epr.load('tempo.DSC')
# scale between -1 and 1
tempo_scaled = tempo.scale_between(-1,1)
print('After scaling : ')
fig,ax = tempo_scaled.plot()
After scaling :
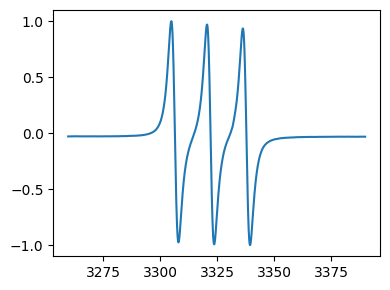
Baseline correction¶
[10]:
# import EPRpy
import eprpy as epr
# load data
tempo = epr.load('tempo.DSC')
# linear baseline correction
tempo_bc = tempo.baseline_correct() # default is linear baseline correction using 10 points at the start and end of the data.
fig,ax = tempo_bc.plot()
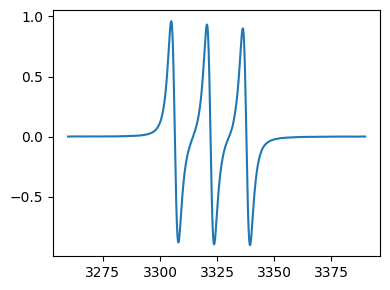
Interactive baseline correction¶
[ ]:
# import EPRpy
import eprpy as epr
# load data needs baseline correction
epr_data = epr.load('data_for_bc.DTA')
# plot the data without bc
fig,ax = epr_data.plot()
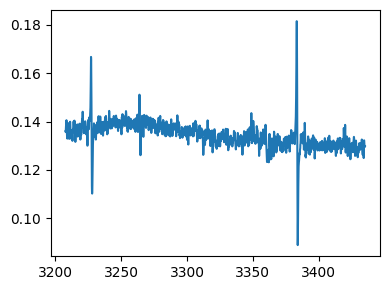
As an example, we baseline correct the spectrum by using a 3° polynomial. For baseline calculation, the points are picked interactively.
[ ]:
# if you are using a jupyter notebook, switch to qt backend for matplotlib
#%matplotlib qt
# to switch back to inline mode:
#%matplotlib inline
[ ]:
epr_data_bc = epr_data.baseline_correct(interactive=True, method='polynomial',order=3)
[ ]:
# plot the baseline corrected spectra
fig,ax = epr_data_bc.plot()
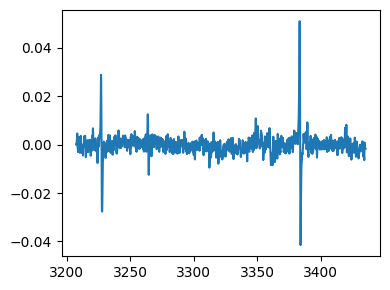
Computing integrals¶
[11]:
# import EPRpy
import eprpy as epr
# load data
tempo = epr.load('tempo.DSC')
# baseline correction and integration
tempo_proc = tempo.baseline_correct()
tempo_proc = tempo_proc.integral()
# plot the absorption signal
fig,ax = tempo_proc.plot()
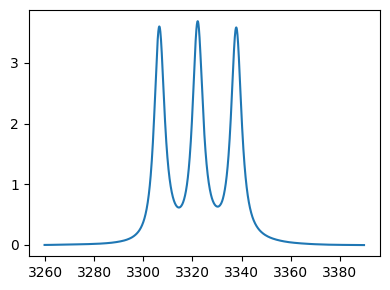
Selecting a region¶
For this example, we assume that the desired region is the low field hyperfine line.
[12]:
# import EPRpy
import eprpy as epr
# load data
tempo = epr.load('tempo.DSC')
# Plot the data using an array with unity spacing to find corresponding indices
import matplotlib.pyplot as plt
print('Full spectrum : ')
_=plt.plot(tempo.data)
# make a new EprData object corresponding to the desired region
tempo_region = tempo.select_region(range(550,850)) # indices from 0 to 900
Full spectrum :
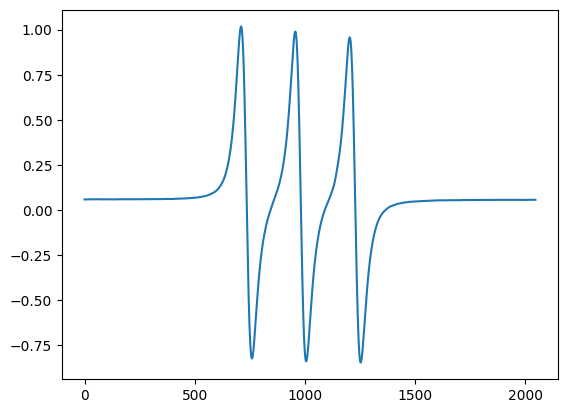
[13]:
# selected region, note that the the new EprData object tempo_region only has the data corresponding to this region
print('Selected region : ')
fig,ax = tempo_region.plot()
Selected region :
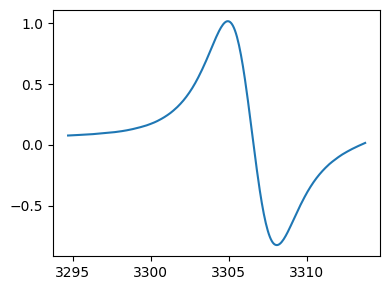
History of data operations¶
EPRpy allows for keeping a log of data operations done on an EprData object, as long as in-built functions are used.
[16]:
# import EPRpy
import eprpy as epr
# load data
tempo = epr.load('tempo.DSC')
# do some operations
tempo_proc1 = tempo.scale_between(-1,1)
tempo_proc2 = tempo_proc1.baseline_correct()
tempo_proc3 = tempo_proc2.integral()
Access history¶
Each
EprData
object stores history of all data operations and the correspondingEprData
object in a list of lists. The first item in each list is the description of the data operation and the second item is the correspondingEprData
object.
[17]:
tempo_proc3.history
[17]:
[['2025-02-03 14:07:02.431224 : Data loaded from tempo.DSC.',
<eprpy.loader.EprData at 0x126d47230>],
['2025-02-03 14:07:02.434587 : Data scaled between -1 and 1.',
<eprpy.loader.EprData at 0x126d473b0>],
['2025-02-03 14:07:02.435971 : Baseline corrected',
<eprpy.loader.EprData at 0x126d473e0>],
['2025-02-03 14:07:02.439949 : Integral calculated',
<eprpy.loader.EprData at 0x126cc3a40>]]
To plot or access a specific
EprData
from the history, simply use indexing. For instance, to view the original data again, plot the first item in history:
[18]:
print(tempo_proc3.history[0][0])
fig,ax = tempo_proc3.history[0][1].plot()
2025-02-03 14:07:02.431224 : Data loaded from tempo.DSC.
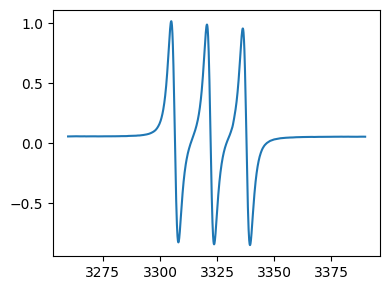
[19]:
print(tempo_proc3.history[2][0])
fig,ax = tempo_proc3.history[2][1].plot()
2025-02-03 14:07:02.435971 : Baseline corrected
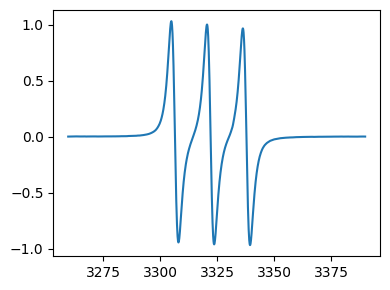
Data (2D)¶
## Importing EPRpy
[16]:
import eprpy as epr
Loading data¶
[17]:
## TEMPO radical monitored as a function of time
## For some 2D datasets, a .GF file must also be present in the same folder.
tempo2d = epr.load('tempo_time.DSC')
[20]:
tempo2d.data.shape # this dataset corresponds to 48 time values (tempo2d.y) and 1024 field points (tempo2d.x)
[20]:
(48, 1024)
[21]:
tempo2d.y # time in seconds
[21]:
array([ 0. , 1533.1 , 3065.64, 4598.25, 6130.95, 7663.46,
9196.02, 10728.54, 12261.1 , 13793.49, 15326.14, 16858.79,
18391.58, 19924.32, 21457.01, 22989.5 , 24521.66, 26054.41,
27586.94, 29119.64, 30652.35, 32184.94, 33717.75, 35250.49,
36783.1 , 38315.81, 39848.56, 41381.3 , 42913.97, 44446.53,
45978.94, 47511.65, 49044.47, 50576.93, 52109.66, 53642.3 ,
55175.05, 56707.73, 58240.42, 59773.14, 61305.37, 62837.76,
64370.26, 65902.73, 67435.12, 68967.26, 70499.68, 72031.99],
dtype='>f8')
[22]:
tempo2d.x # field values in Gauss
[22]:
array([3273.65 , 3273.74658203, 3273.84316406, ..., 3372.26025394,
3372.35683597, 3372.453418 ])
Plotting¶
Stacked plot¶
[4]:
fig,ax = tempo2d.plot(spacing=5)
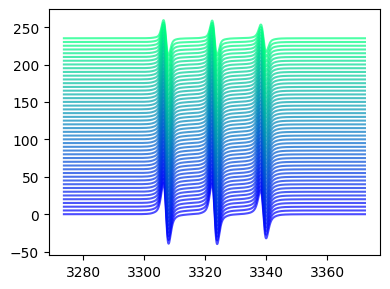
Superimposed plot¶
[5]:
fig,ax = tempo2d.plot(plot_type='superimposed')
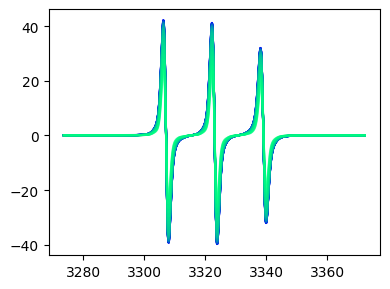
Pseudocolor plots¶
[6]:
fig,ax = tempo2d.plot(plot_type='pcolor')
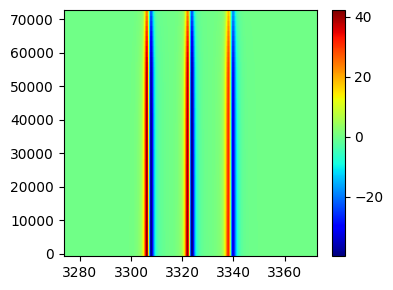
Surface 3D plot¶
[8]:
fig,ax = tempo2d.plot(plot_type='surf')